Learn the Basics of Python: Your Guide to Getting Started
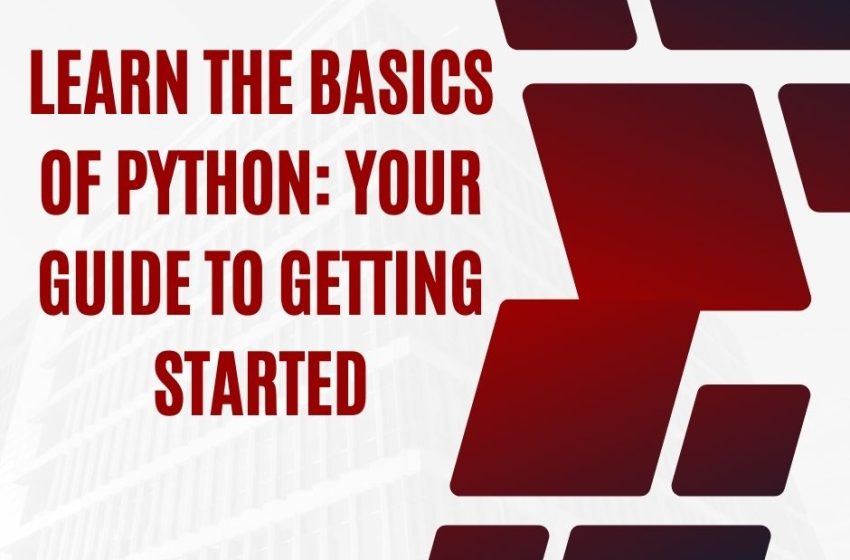
Python is a versatile, high-level programming language known for its readability and simplicity. It’s widely used in web development, data analysis, artificial intelligence, scientific computing, and many other fields. Learning Python can open doors to numerous opportunities in tech.
Why Learn Python?
-
Beginner-Friendly: Python’s syntax is straightforward and easy to learn, making it an excellent choice for beginners.
-
Versatile Applications: From web development to data science, Python is used in various domains.
-
Large Community: Python has a vast community, offering extensive resources, libraries, and frameworks to support your learning journey.
-
High Demand: Python developers are in high demand, with opportunities in many tech industries.
Basic Concepts of Python
-
Syntax and Indentation: Python uses indentation to define code blocks, making the code more readable.
python
if True:
print(“Hello, World!”)
-
Variables and Data Types: Learn about different data types like integers, floats, strings, and lists.
python
x = 5 # Integer
y = 3.14 # Float
name = “John” # String
fruits = [“apple”, “banana”, “cherry”] # List
-
Control Structures: Understand how to use if-else statements, loops, and functions.
python
for fruit in fruits:
print(fruit)
def greet(name):
return f”Hello, {name}!”
print(greet(“Alice”))
-
Functions and Modules: Learn to write reusable code with functions and organize code using modules.
python
def add(a, b):
return a + b
import math
print(math.sqrt(16))
-
File Handling: Understand how to read from and write to files.
python
with open(“example.txt”, “w”) as file:
file.write(“Hello, Python!”)
-
Error Handling: Learn to handle exceptions to make your code robust.
python
try:
x = 10 / 0
except ZeroDivisionError:
print(“You can’t divide by zero!”)
Getting Started with Python
-
Install Python: Download and install Python from the official website.
-
Set Up a Development Environment: Choose an Integrated Development Environment (IDE) like PyCharm, VS Code, or Jupyter Notebook.
-
Follow Tutorials and Courses: Utilize online resources like Codecademy, Coursera, or freeCodeCamp to learn Python step-by-step.
-
Practice Coding: Work on small projects and challenges on platforms like LeetCode, HackerRank, or Codewars.
Popular IDEs for Python Development: Choosing the Best Tool
What is an IDE?
An Integrated Development Environment (IDE) is a software application that provides comprehensive facilities to programmers for software development. It typically includes a code editor, debugger, and build automation tools. Choosing the right IDE can enhance productivity and make coding more enjoyable.
Top IDEs for Python Development
-
PyCharm:
-
Features: Intelligent code editor, powerful debugger, integrated unit testing, version control integration.
-
Pros: Rich feature set, great for large projects, strong support for web development frameworks like Django and Flask.
-
Cons: Can be resource-intensive.
-
-
Visual Studio Code (VS Code):
-
Features: Lightweight, customizable with extensions, built-in Git integration, integrated terminal.
-
Pros: Highly extensible, free, active community, supports multiple languages.
-
Cons: Requires customization for a full-featured experience.
-
-
Jupyter Notebook:
-
Features: Interactive computing environment, supports live code, equations, visualizations, and narrative text.
-
Pros: Excellent for data science and machine learning, interactive data visualization, supports multiple languages via kernels.
-
Cons: Not ideal for large-scale software development.
-
-
Spyder:
-
Features: Scientific Python Development Environment, includes powerful editing, interactive testing, debugging, and introspection tools.
-
Pros: Great for data science, integrates well with Anaconda, includes powerful tools for scientific computing.
-
Cons: Limited support for web development frameworks.
-
-
Atom:
-
Features: Hackable text editor, smart autocompletion, file system browser, built-in package manager.
-
Pros: Highly customizable, strong community support, Git integration.
-
Cons: Can be slow with large files or projects.
-
Choosing the Right IDE
-
For Beginners: Start with VS Code or Jupyter Notebook due to their simplicity and ease of use.
-
For Data Scientists: Jupyter Notebook and Spyder offer the best tools for data analysis and visualization.
-
For Web Developers: PyCharm provides robust support for web development frameworks and complex projects.
-
For Customization: VS Code and Atom allow extensive customization to fit your specific workflow.
Conclusion
Learning Python and choosing the right IDE are critical steps in your development journey. Whether you are a beginner or an experienced developer, the right tools and resources can significantly enhance your productivity and coding experience. Explore different IDEs, find what works best for you, and start building your Python projects today.